String
In Python, strings are used to store text or alphanumeric data. Strings are represented by enclosing the data in single quotes ('
) or double quotes ("
).
Strings are a sequence type, meaning their characters are ordered and can be accessed using indices.
Strings belong to the built-in str class in Python.
You cannot use single or double quotes for multi-line text. It will cause a SyntaxError
# Using double quotes
string1 = "Hello, Python!"
# Using single quotes
string2 = 'Hello, World!'
print(string1, type(string1))
print(string2, type(string2))
Python allows you to store string data in two main ways. Those are
- Single-line string
- Multi-line string
- These are strings that are written on one line. We can use either single quotes or double quotes.
- We can`t use single or double quotes to organize multi-line string data.
# single quotes
name = 'Hello Python'
print(name)
# double quotes
var = 'Hello Cedlearn'
print(var)
# Error
str = " hello
Python"
The Multi Line string data is one , which is enclosed within tripple single Quotes or tripple double quotes.
-
Multi-line strings can be created using either triple single quotes (
'''
) or triple double quotes ("""
). These strings can span multiple lines - String literals that span multiple lines for improved readability.
addr1="""Guido van Rossum
HNO:3-4, Red seaside
Python Software Foundation
Centrum Wiscunde Informatica
Nether Lands """
print(addr1,type(addr1))
On string, we can perform the two types of operations.
- Indexing
- Slicing
- The process of obtaining an element by giving a string object and passing a valid index value.
- The index can either be Positive or Negative.
- The positive index starts with 0 and the negative index starts with -1.
- If we enter a valid index then get values at that index. If you enter an invalid index then get IndexError
# Positive index
my_str = "Python"
print(my_str[0])
print(my_str[1])
print(my_str[2])
print(my_str[3])
# IndexError
my_str = "Python"
print(my_str[10])
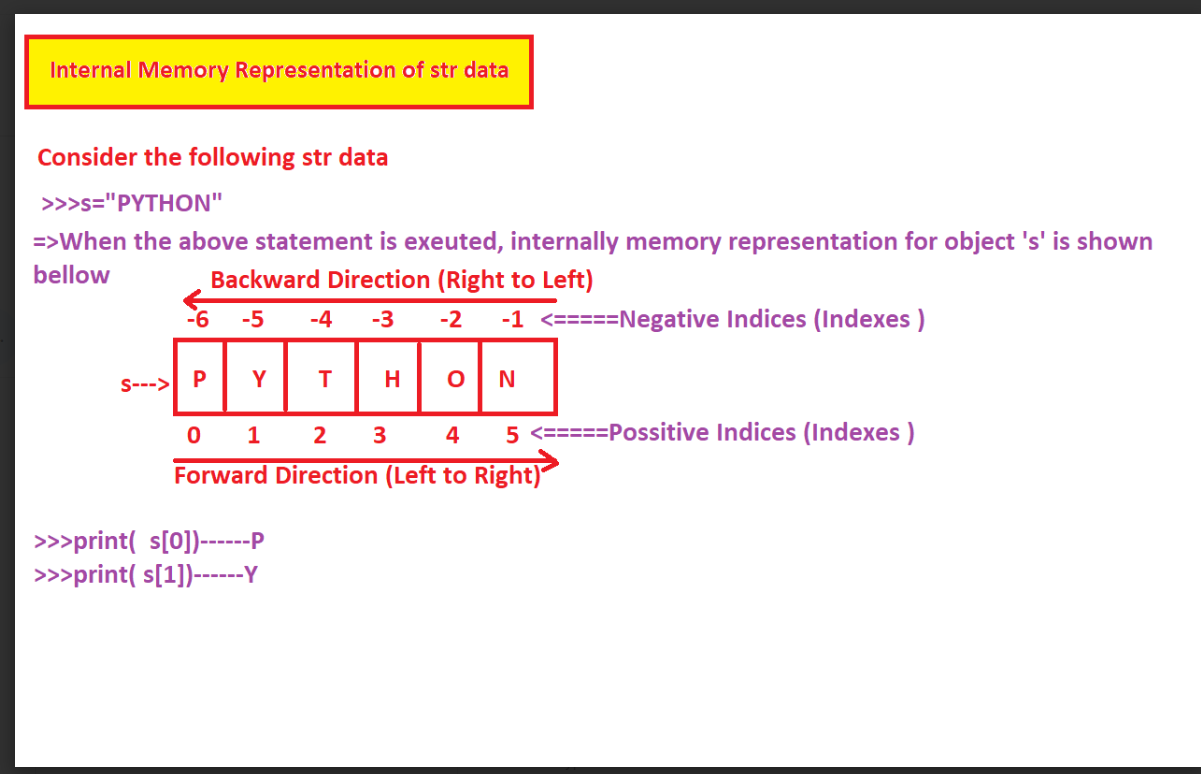
- The process of obtaining the range of characters or sub-strings of the main string is called slicing.
-
The syntax
string[Begin:End]
extracts characters from the "Begin" index (inclusive) to the "End" index - 1 (exclusive). -
If
Begin Index >= End Index
, the result is an empty output (''
) .
# slicing using +ve index
my_str = "Python"
print(my_str[2:4])
print(my_str[1:6])
print(my_str[4:2]) # empty
# Slicing with -ve index
my_str = "Python"
print(my_str[-4:-2])
print(my_str[-6:-1])
print(my_str[-10:-1]) # Python
print(my_str[-1:-5])# empty
-
If the Begin Index and End Index are not specified, Python assumes the End Index as
len(strobj) - 1
.
-
This syntax retrieves characters starting from the Begin Index to the last character of the string object (
len(strobj) - 1
).
#
s="PYTHON"
print(s[2:])-----------THON
print(s[1:])----------YTHON
print(s[4:])-------ON
print(s[-2:])---------ON
print(s[-6:])-------PYTHON
print(s[0:])--------PYTHON
print(s[-2:])-------ON
print(s[-5:])-------YTHON
-
If the Begin Index is not specified and the End Index is specified, Python assumes the Begin Index as the initial index (
0
).
-
This syntax retrieves characters from the initial index (
0
) toEnd Index - 1
of the string object.
s="PYTHON"
print(s[ : 5] )------------PYTHO
print(s[ : 5])------------PYTHO
print(s[ : 2])-----------PY
print(s[ : 3])----------PYT
print(s[ :-3])---------PYT
print(s[ :-4])--------PY
print(s[ :-6])-----empty
-
If neither the Begin Index nor the End Index is specified, Python assumes the Begin Index as the initial index (
0
) and the End Index aslen(strobj) - 1
.
- This syntax retrieves the complete data of the string object.
s="PYTHON"
>>> print(s[ : ])--------PYTHON
>>> print(s)------PYTHON
-
The syntax
strobj[BEGIN:END:STEP]
allows slicing with an optional step value, where the default value ofSTEP
is1
, processing the string in a forward direction.
-
If
STEP
is positive, it slices characters fromBEGIN
toEND - 1
in a forward direction (left to right).
-
If
STEP
is negative, it slices characters fromBEGIN
toEND + 1
in a backward direction (right to left).
-
When slicing forward, an
END
value of0
results in no output.
-
When slicing backward, an
END
value of-1
results in no output.
# Slicing with step value
s="PYTHON"
print(s[0:6:2])----------PTO
print(s[0:6:3])---------PH
print(s[2:6:3])---------TN
print(s[-6:-3:2] )-------PT
print(s[ :4:2])--------PT
print(s[3: :2])----------HN
print(s[ : : 2])--------PTO
print(s[ : : 1])--------PYTHON
s="PYTHON"
print(s[2:6:-1])------- No output
print(s[6:2:-1])--------NOH
print(s[5:0:-2])-------NHY
print(s[::-2])--------NHY
print(s[::-1])----------NOHTYP
s="NAYAN"
print(s[::1])------NAYAN
print(s[::-1])----------NAYAN
print(s[:0:1])---No Output
print(s[:-1:-1])-----No Output