Operators
- An operator is a symbol used to perform specific operations on given data. When two or more variables or objects are connected with an operator is called Expression.
- The main purpose of an operator is to perform operations on variables or data to achieve a specific result.
In Python 7 different types of operators are there. They are
- Arithmetic Operator
- Assignment Operator
- Relational Operators
- Logical Operators
- Bitwise Operators
- Membership Operators
- Identity Operators
Arithmetic operators are symbols that denote mathematical operations such as addition, subtraction, multiplication, and division. When two or more variables or objects are combined using arithmetic operators, the resulting combination is called an arithmetic expression.
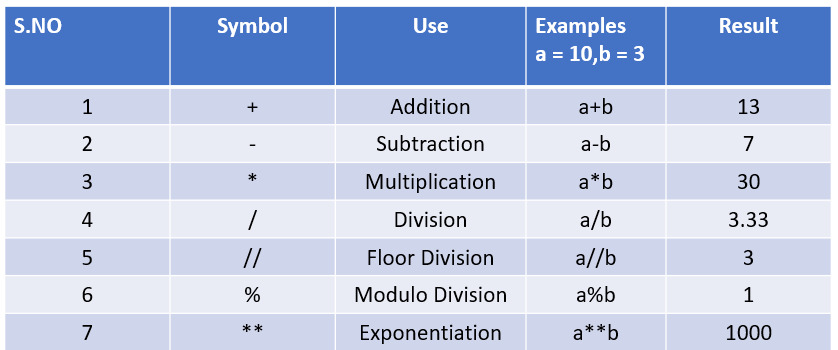
- The symbol for the assignment operator is =
- The main purpose of the Assignment operator is To transfer the RHS value to the LHS Variable.
- We can use the Assignment operators in two ways. There are
- Single Line Assignment Operator.
- Multi-Line Assignment Operator
I. Single Line Assignment Operator
This Operator transfers single values from RSH to a single Variable of LHS.
Syntax :
varname = value
II. Multi-Line Assignment Operator.
This operator transfers multiple values from RHS to Multiple Variables of LHS.
Syntax:
var1,var2,var3...varn = val1,val2,val3..... valn
Here are the values and variables(LHS and RHS) should be the same.
- Relational operators are used to compare two values, helping determine their relationship (e.g., equal, greater, or less).
- When two or more values or variables are connected using relational operators, it forms a relational expression.
-
The result of a relational expression is always either
True
orFalse
, which aids in decision-making processes.
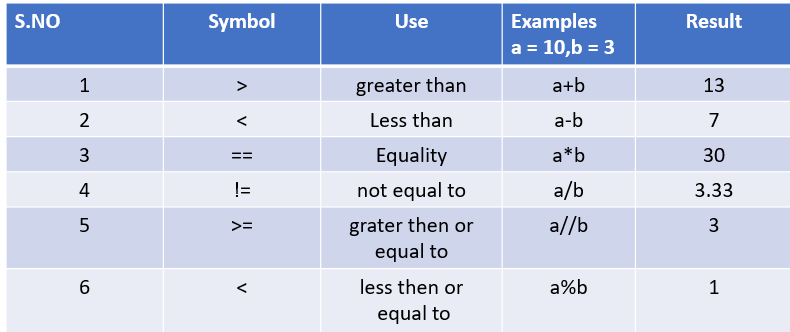
- Logical operators are used to combine two or more relational expressions to evaluate a collective condition.
-
Logical expressions, formed by connecting relational expressions with logical operators, result in either
True
orFalse
. They are also known as compound conditions. - There are three types of logical operators, used to structure complex conditions in decision-making.
- and
- or
- not
1. and Operator
-
The Logical AND Operator evaluates to
True
only if all the conditions in the expression areTrue
; otherwise, it evaluates toFalse
. -
In the case of the 'and' operator, if it is connected with multiple relational expressions and the first relational expression evaluates to
False
, the remaining relational expressions will not be evaluated, and the result will be consideredFalse
."
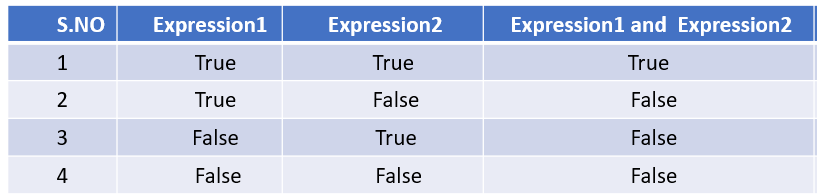
2. or Operator
-
The Logical or Operator evaluates to
True
only if any of the conditions in the expression areTrue
; otherwise, it evaluates toFalse
. -
"In the case of the 'or' operator, if it is connected with multiple relational expressions and the first relational expression evaluates to
True
, the remaining relational expressions will not be evaluated, and the result will be consideredTrue
."
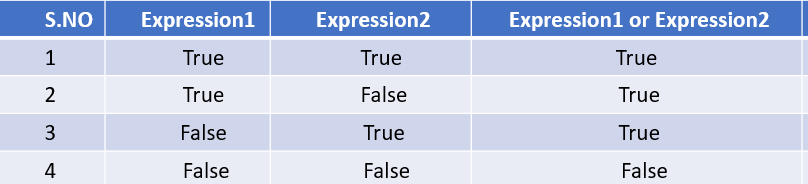
3. not Operator
- This operator gives the opposite result of a Boolean value.

Bitwise Operators apply only to integer data and not to floating-point values because floating-point numbers have varying precision.
The purpose of Bitwise Operators is as follows:
- They convert integer data internally into binary format and perform binary operations bit by bit.
- The final result is always displayed in decimal number format.
Since the operations are performed bit by bit, these operators are named Bitwise Operators.
In Python programming, the following bitwise operators are available.
-
Bitwise Left Shift Operator (
<<
)
-
Bitwise Right Shift Operator (
>>
)
-
Bitwise AND Operator (
&
)
-
Bitwise OR Operator (
|
)
-
Bitwise Complement Operator (
~
)
-
Bitwise XOR Operator (
^
)
1. Bitwise Left shift Operator(<<)
- The concept of the bitwise left shift operator is to shift the specified number of bits to the left by adding zeros to the right side. The number of zeros added is equal to the specified number of bits.
- Syntax:- varname = GivenData << No.of Bits
2. Bitwise Right shift Operator(>>)
- The bitwise right shift operator shifts the specified number of bits to the right by adding zeros to the left side. The number of zeros added is equal to the specified number of bits.
- Syntax:- varname = GivenData >> No.of Bits
3. Bitwise and Operator( & )
The Bitwise AND operator compares each bit of the two inputs and returns
-
1
if both bits are1
. -
0
otherwise. - Syntax:- varname = value1 & value2
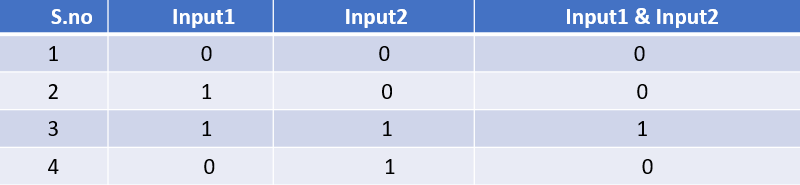
4. Bitwise or Operator( | )
The Bitwise or operator compares each bit of the two inputs and returns
-
1
if anyone bit is1
. -
0
otherwise. - Syntax:- varname = value1 | value2
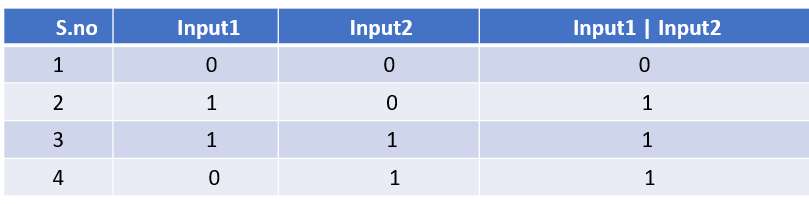
5. Bitwise complement Operator( ~ )
-
bitwise complement operator (
~
) inverts the bits of a number. It flips all1
s to0
s and all0
s to1
s.
-
In the above code the binary representation of
5
is00000101
. -
The complement is
11111010
(in binary)-6
in decimal (2's complement representation). - The most significant bit (MSB) acts as the sign bit.
-
0
Represents a positive number. -
1
: Represents a negative number.
- Positive numbers are stored directly in binary format.
-
Negative numbers are stored in 2's complement form, which includes flipping all bits and adding
1
.
- Syntax:- varname = ~value
6. Bitwise xor Operator(^)
-
The Bitwise XOR (Exclusive OR) Operator performs a comparison of bits from two numbers. It returns
1
if the corresponding bits of the two numbers are different and0
if they are the same.
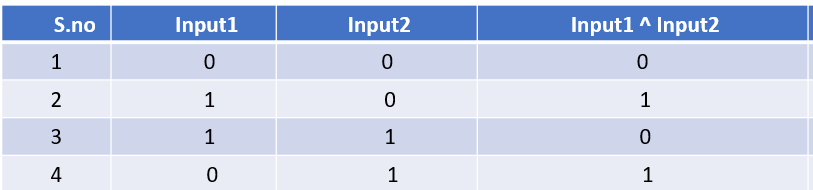
- The purpose of Membership Operators is to check if a specified value is present in an iterable object.
- An iterable object is an object that contains multiple elements, such as sequence types (strings, lists, tuples), sets, and dictionaries.
- In Python, there are two types of Membership Operators.
- in
- not in
1. in Operator
-
If the value is present in the Iterable_Object, the expression returns
True
otherwise, it returnsFalse
.
-
Here, the variable varname will contain either
True
orFalse
, and its type is bool. - Syntax :- varname = value in itrable_obect
2. not in Operator
-
If the value is present not in the Iterable_Object, the expression returns
True
otherwise, it returnsFalse
.
-
Here, the variable varname will contain either
True
orFalse
, and its type is bool. - Syntax :- varname = value not in itrable_obect
- The purpose of Identity Operators is to compare the memory address of objects.
-
To get the memory address of an object/variable, we use the
id()
function. - In Python, there are two Identity Operators.
- is
- is not
1. is Operator:
-
The
is
operator checks if two variables reference the same object in memory. It returnsTrue
if they do, andFalse
otherwise. - Syntax:- var1 is var2
2. is not Operator
-
The
is not
operator checks if two variables reference the different objects in memory. It returnsTrue
if they do, andFalse
otherwise.
- Syntax:- var1 is not var2